Download
User Rating: 4/5 ( 1 votes)
This Coverflow project seeks to create a fully functional 'CoverFlow' effect using a combination of jQuery, jQuery UI components and CSS3 transforms.
Features:
-
highly optimized animations
-
auto-detects browser rendering capabilities
-
provides a 3D rendering mode with a 2D fallback
-
momentum swipe support (based on external libs, offically supported are jQuery mobile and Hammer.js)
-
mousewheel support
-
legacy browser support down to Internet Explorer 8
-
with good code coverage
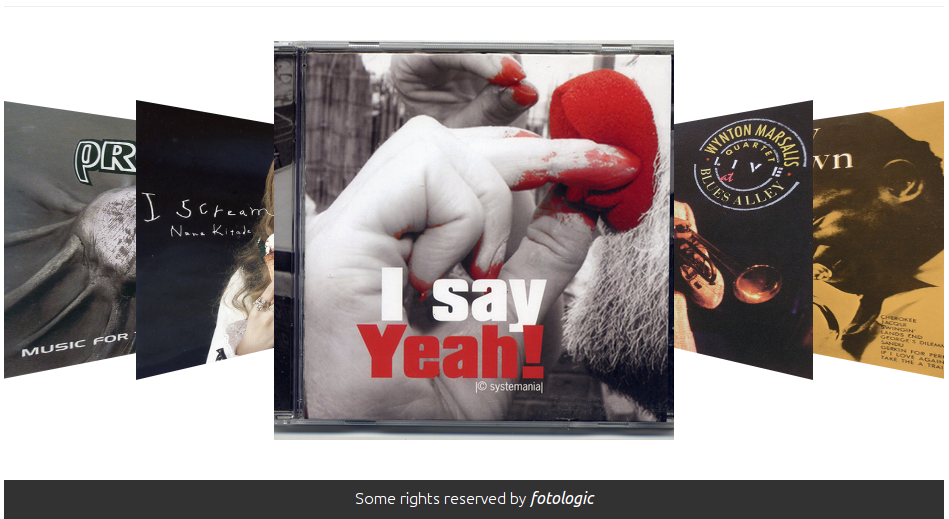
1. INCLUDE CSS AND JS FILES
<!-- put this in your html header -->
<link rel="stylesheet" type="text/css" href="coverflow.css" />
<script src="jquery.min.js"></script>
<!-- include coverflow after all dependencies-->
<script src="coverflow.min.js"></script>
2. HTML
<div id="coverflow">
<!-- these children will be part of the coverflow -->
<div class="something_fancy"></div>
<img src=".." />
<picture><source .. /></picture>
</div>
3. JAVASCRIPT
$(function() {
// and kick off
$('#coverflow').coverflow();
});
4. OPTIONS
-
items (string):
Any valid jQuery Selector. Default any child element of your coverflow container.
-
active (int >=0):
active item index on initialisation, zero based. Default the first item gets selected.
-
duration (int):
animation duration in ms, default 400.
-
overlap (float):
Configures items overlapping. Value between 0 and 1. Default 0.3.
-
scale (float):
Configures scaling of inactive items. Value between 0 and 1. Default 0.7.
-
angle (int):
Angle of item rotation. Defaults to 45°
Only active when 3D mode is available
-
perspectiveY (int):
Configure perspective angle. Default 45
Only active when 3D mode is available
-
easing (string):
Easing used for animation. Defaults to ‘easeOutQuint’.
Available easing functions:
The following easings (included in jQuery-ui) are not available as cubic bezier timing functions in CSS3:
-
easeInQuad
-
easeInCubic
-
easeInQuart
-
easeInQuint
-
easeInSine
-
easeInExpo
-
easeInCirc
-
easeInBack
-
easeOutQuad
-
easeOutCubic
-
easeOutQuart
-
easeOutQuint
-
easeOutSine
-
easeOutExpo
-
easeOutCirc
-
easeOutBack
-
easeInOutQuad
-
easeInOutCubic
-
easeInOutQuart
-
easeInOutQuint
-
easeInOutSine
-
easeInOutExpo
-
easeInOutCirc
-
easeInOutBack
-
easeInElastic
-
easeOutElastic
-
easeInOutElastic
-
easeInBounce
-
easeOutBounce
-
easeInOutBounce
-
trigger (plain object):
automatic event bindings you may want to customize. Your options are:
- itemfocus (*disabled by default, competes with swipe support*)
- itemclick
- mousewheel
- swipe
5. METHODS
-
select( {Number} index/{jQuery} item ):
Returns a boolean (selection success). Pass an item index (zero based) or any valid jQuery coverflow item. So these expressions are equivalent:
$('#coverflow' ).coverflow( 'select', 2 );
var el = $('#coverflow' ); el.coverflow( 'select', el.children().eq(2) ) );
-
next()
$('#coverflow' ).coverflow( 'prev' );
Returns a boolean (selection success). Selects next/previous item.
-
prev()
$('#coverflow' ).coverflow( 'prev' );
Returns a boolean (selection success). Selects next/previous item.
6. EVENTS
Plugin Events
Event data
The ui object passed to any coverflow event handler consists of 2 members:
-
active: jQuery object representing the current active item
-
index: zero based index pointing to active item