Download
User Rating: 0/5 ( 0 votes)
What is it?
Tinycircleslider is a circular slider / carousel. That was built to provide webdevelopers with a cool but subtle alternative to all those standard carousels. Tiny Circleslider can blend in on any wepage. It was built using the javascript jQuery library.
Features
-
IOS and Android support.
-
AMD, Node, requirejs and commonjs support.
-
Supports sliding by thumb or pager
-
A interval can be set to slide automaticaly every given milliseconds
-
Can be set to snap to a page
-
Option to fade dots when dragging is done
-
Size(radius) of the slider can be set.
-
Can fire a callback after every move.
-
Easy customizable
-
Lightweight
-
Source is on GitHub
Examples
$(document).ready(function()
{
$("#circleslider2").tinycircleslider({
interval : true
, dotsSnap : true
});
});
Note: There is no lightbox natively in tiny circleslider for this example fancybox was used.
$(document).ready(function()
{
$("#circleslider3").tinycircleslider({
dotsSnap : true
, radius : 170
, dotsHide : false
});
$("#circleslider3").find("a").fancybox();
});
To get a better understanding of how it all comes together I made a (corny) styled example. If someone can make a nicer design it would be greatly appreciated :)
$(document).ready(function()
{
$("#circleslider4").tinycircleslider({
dotsSnap : true
, radius : 215
, dotsHide : false
, interval : true
});
});
Initialize options
A list of all the available options and there default value
-
interval : false -- move to another block on intervals.
-
intervalTime : 3500 -- time between intervals.
-
dots : true -- automatic placement of dots or use predefined location on slide.
-
dotsSnap : false -- shows dots when user starts dragging and snap to them.
-
dotsHide : true -- fades out the dots when user stops dragging.
-
lightbox : false -- when you have links with a lightbox attached this most be true for normal links to work correctly this must be false.
-
radius : 140 -- Used to determine the size of the circleslider
The image below displays how you calculate the radius of your circleslider. The center of your slider to the center of the outside circle is your radius.
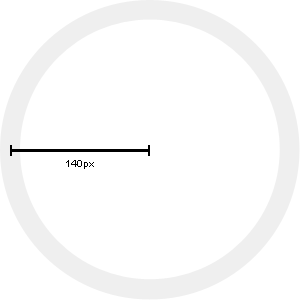
Properties
$(document).ready(function()
{
// You can access the methods and properties of your
// slider instance straight after initialization.
//
$('#box').tinycircleslider();
// This part you can do from whatever place in your
// code as long as the slider allready is initialized.
//
var box = $('#box').data("plugin_tinycircleslider");
// Now you have access to all the methods and properties.
//
// box.update();
// console.log(box.slideCurrent);
//
// etc..
});
-
slideCurrent -- The index of the current slide.
-
angleCurrent -- The current angle of the thumb.
Methods
-
start -- Start the interval.
-
stop -- Stop the interval.
-
move -- Move to specific slide. The only param you can supply is the index of the slide.
Events
$(document).ready(function()
{
var $box = $('#box');
var box = $box.tinycircleslider();
$box.bind("move", function()
{
console.log("do something on every move to a new slide");
});
});
-
move -- The move event will trigger when the carousel slides to a new slide.